API Access Process
Default rule
- Use
HTTPS
as the transport protocol, defaultPOST
request API
follows theREST
style and usesJSON
as the packet transfer protocol by default- Pay attention to UrlEncode
- Interface restriction: The default number of RequestBody/ResponseBody is less than 500 and smaller than 1Mb
- The default timeout interval is 60s
Flow of communication
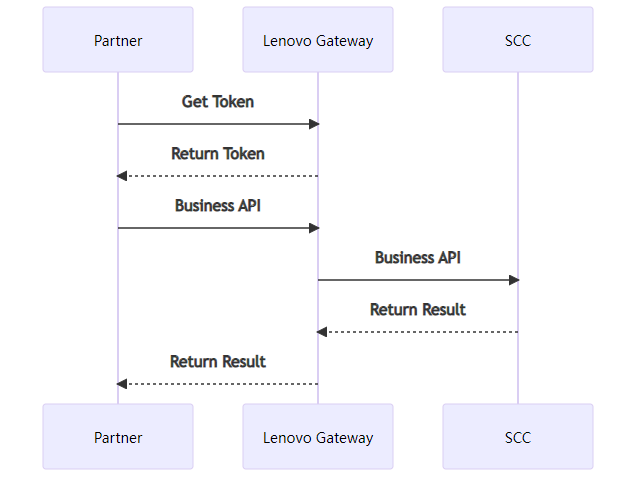
Access Preparation
This access | Precautions |
---|---|
first access | Lenovo assigns secure clientId, clientSecret, and interface permissions |
Not first access | Provides a history clientId for Lenovo to add new interface permissions |
Environment Information
Environment | URL | clientId | clientSecret |
---|---|---|---|
Test | https://api-cn-t.lenovo.com/uat | Inform after application | Inform after application |
Prod | https://api-cn.lenovo.com | Inform after application | Inform after application |
Get Token Authentication
Token use the client_credentials mechanism of Oauth2 and have an expiration mechanism. Note that tokens should be cached and updated, and frequent refreshes should be avoided
Environment | Authentication URL |
---|---|
Test | https://api-cn-t.lenovo.com/uat/token |
Prod | https://api-cn.lenovo.com/token |
Request Parameters
position | field | required | description | sample |
---|---|---|---|---|
Header | Content-Type | Y | fixed value | application/x-www-form-urlencoded |
Header | Authorization | Y | Base64(consumer-key:consumer-secret) | Basic SHlFdUFzUm80WjlLdEJLRGpJNUdhOW1mVGZJYTpnZWdDTVFENkZtYlBuWDU2RjNzTW1VYWhtVVVh |
Parameter | grant_type | Y | fixed value | client_credentials |
Response Parameters
position | field | required | type | description | sample |
---|---|---|---|---|---|
Body | access_token | Y | string | Token | aaf0d4d6-c541-34cd-a4e0-03da1cc4019d |
Body | scope | Y | string | scope | am_application_scope default |
Body | token_type | Y | string | token_type | Bearer |
Body | expires_in | Y | number | Validity period (seconds) | 600 |
- curl Request Example
bash
curl -k -d "grant_type=client_credentials" -u clientId:clientSecret https://api-cn-t.lenovo.com/uat/token
bash
curl -k -d "grant_type=client_credentials" \
-H "Authorization: Basic Base64(clientId:clientSecret)" \
https://api-cn-t.lenovo.com/uat/token
- Java Request Example
java
import org.apache.oltu.oauth2.client.OAuthClient;
import org.apache.oltu.oauth2.client.URLConnectionClient;
import org.apache.oltu.oauth2.client.request.OAuthClientRequest;
import org.apache.oltu.oauth2.client.response.OAuthAccessTokenResponse;
import org.apache.oltu.oauth2.client.response.OAuthJSONAccessTokenResponse;
import org.apache.oltu.oauth2.common.exception.OAuthProblemException;
import org.apache.oltu.oauth2.common.exception.OAuthSystemException;
import org.apache.oltu.oauth2.common.message.types.GrantType;
public static OAuthAccessTokenResponse getToken(String clientId, String clientSecret, String oauth2Url) {
try {
OAuthClientRequest accessTokenRequest = OAuthClientRequest.tokenLocation(oauth2Url)
.setGrantType(GrantType.CLIENT_CREDENTIALS)
.setClientId(clientId)
.setClientSecret(clientSecret)
.buildQueryMessage();
OAuthClient client = new OAuthClient(new URLConnectionClient());
OAuthAccessTokenResponse oauthResponse = client.accessToken(accessTokenRequest, OAuthJSONAccessTokenResponse.class);
return oauthResponse;
} catch (OAuthSystemException | OAuthProblemException e) {
log.error("Oauth2Utils getToken Error", e);
}
return null;
}
- Response Example
json
{
"access_token": "aaf0d4d6-c541-34cd-a4e0-03da1cc4019d",
"scope": "am_application_scope default",
"token_type": "Bearer",
"expires_in": 600
}
Token Usage Mode
For all service interfaces, the request Header must carry a Token to ensure security
Common request parameters
position | field | description | sample |
---|---|---|---|
Header | Authorization | Bearer + " " + Token | Bearer aaf0d4d6-c541-34cd-a4e0-03da1cc4019d |